Snippet
Update Google Sheets
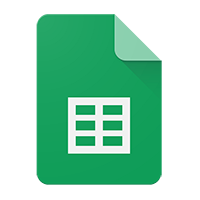
Send submitted form data to a Google Sheet
I am using `react-hook-form` to build a simple form. Once a user submits the form, the data will be inserted into my Google Sheet.
1// /lib/googleSheet.js
2const { google } = require('googleapis')
3const sheets = google.sheets({
4 version: 'v4',
5 auth: process.env.GOOGLE_API_KEY,
6})
7
8const appendToSheet = async (data) => {
9 const sheetId = 'process.env.NEXT_PUBLIC_GOOGLE_SHEET_ID'
10 const range = 'Sheet1!A:A' //the range of the sheet you want to append to
11 const valueInputOption = 'USER_ENTERED'
12 const insertDataOption = 'INSERT_ROWS'
13 const resource = {
14 values: [Object.values(data)],
15 }
16
17 return sheets.spreadsheets.values.append({
18 spreadsheetId: sheetId,
19 range,
20 valueInputOption,
21 insertDataOption,
22 resource,
23 })
24}
25
26// /api/submit-sheet.js
27export default async (req, res) => {
28 try {
29 const { data } = req.body
30 await appendToSheet(data)
31 res.status(200).json({ message: 'Success' })
32 } catch (error) {
33 res.status(500).json({ message: 'Error' })
34 }
35}
36
1// in your form component
2import useForm from 'react-hook-form'
3
4const Form = () => {
5 const { register, handleSubmit } = useForm()
6
7 const onSubmit = async (data) => {
8 try {
9 const res = await fetch('/api/submit', {
10 method: 'POST',
11 headers: { 'Content-Type': 'application/json' },
12 body: JSON.stringify({ data }),
13 })
14 const json = await res.json()
15 console.log(json.message)
16 } catch (error) {
17 console.log(error)
18 }
19 }
20
21 return (
22 <form onSubmit={handleSubmit(onSubmit)}>
23 <input
24 type="text"
25 name="name"
26 placeholder="Name"
27 ref={register}
28 />
29 <input
30 type="email"
31 name="email"
32 placeholder="Email"
33 ref={register}
34 />
35 <button type="submit">Submit</button>
36 </form>
37 )
38}
39
40export default Form
41
These components are responsible for creating a form that accepts user input, submitting the form data to an API route `/api/submit-sheet`, which in turn saves the data to a Google Sheet using the `appendToSheet` function.
The `appendToSheet` function is a module that uses the `googleapis` library to interact with Google Sheets API. It appends the data passed to it as a new row to a sheet with ID stored in the `process.env.NEXT_PUBLIC_GOOGLE_SHEET_ID` environment variable.
The `submit-sheet.js` API route receives the data sent by the form and passes it to the `appendToSheet` function to save to Google Sheets. It returns a success message if the data is saved successfully or an error message if the saving process fails.
The `Form` component uses the `react-hook-form` library to manage the form state and validation. When the user submits the form, it sends the form data as a POST request to the `/api/submit-sheet` API route, which handles the saving of data to Google Sheets. The success or error message is logged to the console.