Snippet
Spotify Currently Playing
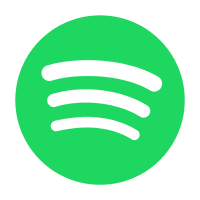
Display the song currently playing on your Spotify account
A useful JavaScript code snippet, using Spotify's APIs to fetch the currently playing track on your Spotify account. This example uses Next.js API Routes.
// /lib/spotify.js
const client_id = process.env.SPOTIFY_CLIENT_ID
const client_secret = process.env.SPOTIFY_CLIENT_SECRET
const refresh_token = process.env.SPOTIFY_REFRESH_TOKEN
const getAccessToken = async () => {
const response = await fetch('https://accounts.spotify.com/api/token', {
method: 'POST',
headers: {
Authorization: `Basic ${Buffer.from(`${client_id}:${client_secret}`).toString('base64')}`,
'Content-Type': 'application/x-www-form-urlencoded'
},
body: new URLSearchParams({
grant_type: 'refresh_token',
refresh_token: refresh_token
})
})
return response.json()
}
export const getNowPlaying = async () => {
const { access_token } = await getAccessToken()
return fetch('https://api.spotify.com/v1/me/player/currently-playing', {
headers: {
Authorization: `Bearer ${access_token}`
}
})
}
// /api/currently-playing.js
import { getNowPlaying } from '#/lib/spotify'
export default async function handler(req, res) {
const response = await getNowPlaying()
if (response.status === 204 || response.status > 400) {
return res.status(200).json({ isPlaying: false })
}
const song = await response.json()
if (song.item === null) {
return res.status(200).json({ isPlaying: false })
}
const isPlaying = song.is_playing
const title = song.item.name
const artist = song.item.artists.map((_artist) => _artist.name).join(', ')
const album = song.item.album.name
const albumImageUrl = song.item.album.images[0].url
const songUrl = song.item.external_urls.spotify
return res.status(200).json({
album,
albumImageUrl,
artist,
isPlaying,
songUrl,
title
})
}
The `spotify.js` file contains a function called `getNowPlaying` that gets the user's access token for Spotify and uses it to fetch the currently playing song using the Spotify Web API. It returns the fetched data as a Promise.
The `currently-playing.js` file is an API route that calls the `getNowPlaying` function from the `spotify.js file`. It then checks whether the response from the API call is valid and contains a playing song. If it does, it returns a JSON object containing information about the song such as its title, artist, album, album image, and URL. If there is no currently playing song, it returns a JSON object indicating that there is no song playing.
Together, these components provide a way to display information about the currently playing song on a user's Spotify account.