Snippet
Back
useScrollDirection Custom Hook
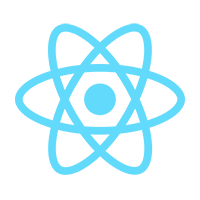
A custom React hook to detect user scroll direction
A useful React custom hook function called `useScrollDirection`, which will detect when the user scrolls up or down. A good use case would be when you want to show/hide the navigation bar.
1// useScrollDirection.js
2import { useEffect, useRef, useState } from 'react'
3
4const THRESHOLD = 0
5
6const useScrollDirection = () => {
7 const [scrollDirection, setScrollDirection] = useState('up')
8
9 const blocking = useRef(false)
10 const prevScrollY = useRef(0)
11
12 useEffect(() => {
13 prevScrollY.current = window.pageYOffset
14
15 const updateScrollDirection = () => {
16 const scrollY = window.pageYOffset
17
18 if (Math.abs(scrollY - prevScrollY.current) >= THRESHOLD) {
19 const newScrollDirection =
20 scrollY > prevScrollY.current ? 'down' : 'up'
21
22 setScrollDirection(newScrollDirection);
23
24 prevScrollY.current = scrollY > 0 ? scrollY : 0
25 }
26
27 blocking.current = false
28 };
29
30 const onScroll = () => {
31 if (!blocking.current) {
32 blocking.current = true
33 window.requestAnimationFrame(updateScrollDirection)
34 }
35 }
36
37 window.addEventListener('scroll', onScroll)
38
39 return () => window.removeEventListener('scroll', onScroll)
40 }, [scrollDirection])
41
42 return scrollDirection
43}
44
45export default useScrollDirection
46
When you call this function, it will return a string value of 'up' or 'down' each time the user scrolls. You can edit the code to your liking and have it return whatever you want.