Snippet
Back
Mailchimp Newsletter Subscribe
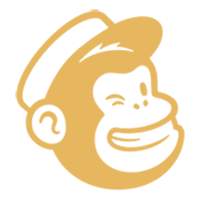
Send form submission data to Mailchimp list
A very basic example of a newsletter signup form, which takes the submitted email address and creates a new member in our Mailchimp list.
1// /api/subscribe.js
2export default async (req, res) => {
3 const { email } = req.body
4 const url = `https://usX.api.mailchimp.com/3.0/lists/${process.env.MAILCHIMP_LIST_ID}/members`
5
6 try {
7 const response = await fetch(url, {
8 method: 'POST',
9 headers: {
10 'Content-Type': 'application/json',
11 'Authorization': `apikey ${process.env.MAILCHIMP_API_KEY}`
12 },
13 body: JSON.stringify({
14 email_address: email,
15 status: 'subscribed'
16 })
17 });
18 const data = await response.json()
19 res.status(200).json({ message: 'Successfully subscribed', data })
20 } catch (error) {
21 res.status(400).json({ message: 'Failed to subscribe', error })
22 }
23}
24
1// in your component
2const handleSubmit = async (e) => {
3 e.preventDefault()
4 const email = e.target.elements.email.value
5 try {
6 const response = await fetch('/api/subscribe', {
7 method: 'POST',
8 headers: {
9 'Content-Type': 'application/json'
10 },
11 body: JSON.stringify({ email }),
12 })
13 const data = await response.json()
14 // handle the response data
15 } catch (error) {
16 // handle the error
17 }
18}
19
20return (
21 <form onSubmit={handleSubmit}>
22 <input type="email" name="email" placeholder="Enter your email" required />
23 <button type="submit">Subscribe</button>
24 </form>
25)
26
In the 2nd code block for the component, we are using the fetch function to make a POST request to the `/api/subscribe.js` route with a JSON payload containing the user's email address. The email address is extracted from the form data using the e.target.elements.email.value method, where `e` is the event object passed to the handleSubmit function.
In the handleSubmit function, we are also handling the response by calling response.json() and handling the data returned. Of course this is very basic, so you will need to tweak it to your liking.