Snippet
Spotify Top Tracks
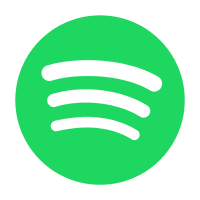
Fetch and display your Spotify top tracks list
This code snippet shows how you can retrieve your Spotify top tracks using Next.js API Routes.
1// /lib/spotify.js
2const client_id = process.env.SPOTIFY_CLIENT_ID
3const client_secret = process.env.SPOTIFY_CLIENT_SECRET
4const refresh_token = process.env.SPOTIFY_REFRESH_TOKEN
5
6const getAccessToken = async () => {
7 const response = await fetch('https://accounts.spotify.com/api/token', {
8 method: 'POST',
9 headers: {
10 Authorization: `Basic ${Buffer.from(`${client_id}:${client_secret}`).toString('base64')}`,
11 'Content-Type': 'application/x-www-form-urlencoded'
12 },
13 body: new URLSearchParams({
14 grant_type: 'refresh_token',
15 refresh_token: refresh_token
16 })
17 })
18
19 return response.json()
20}
21
22export const getTopTracks = async () => {
23 const { access_token } = await getAccessToken()
24
25 return fetch('https://api.spotify.com/v1/me/top/tracks?limit=5&time_range=short_term', {
26 headers: {
27 Authorization: `Bearer ${access_token}`
28 }
29 })
30}
31
1// /api/top-tracks.js
2import { getTopTracks } from '#/lib/spotify'
3
4export default async function handler(req, res) {
5 const response = await getTopTracks()
6 const { items } = await response.json()
7
8 const tracks = items.map((track) => ({
9 artist: track.artists.map((_artist) => _artist.name).join(', '),
10 songUrl: track.external_urls.spotify,
11 title: track.name
12 }))
13
14 res.setHeader(
15 "Cache-Control",
16 "public, s-maxage=86400, stale-while-revalidate=43200"
17 )
18
19 return res.status(200).json(tracks)
20}
21
The `lib/spotify.js` file contains a function `getAccessToken()` that sends a POST request to Spotify's token API endpoint, using the client ID, client secret, and refresh token provided in environment variables. The response contains an access token, which is used to send a GET request to Spotify's API endpoint to retrieve the user's top tracks for a short time period. This function is exported as `getTopTracks()`.
The `api/top-tracks.js` file imports the `getTopTracks()` function from `lib/spotify.js` and uses it to get the user's top tracks for a short time period. The API endpoint returns an array of objects, each containing the name of the artist, the name of the song, and a link to the song on Spotify. The Cache-Control header is set to cache the response for 24 hours and revalidate it after 12 hours.