Snippet
Back
URL Parameters as Objects
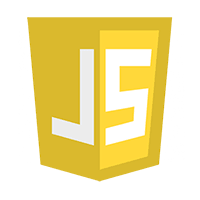
Creates an object containing the parameters of the current URL.
A vanilla JavaScript function to create an object out of URL parameters.
1// add the function
2const getURLParameters = (url) =>
3 (url.match(/([^?=&]+)(=([^&]*))/g) || []).reduce(
4 (a, v) => (
5 (a[v.slice(0, v.indexOf('='))] = v.slice(v.indexOf('=') + 1)), a
6 ),
7 {}
8 )
9
10// call the function
11getURLParameters(window.location.toLocaleString())
12
13// example
14getURLParameters('http://example.com/page?id=1234&name=Bob');
15// {id: '1234', name: 'Bob'}
The above is just a simple example of getting the URL parameters and returning them as an object. In this example we are:
Use String.prototype.match() with an appropriate regular expression to get all key-value pairs.
Use Array.prototype.reduce() to map and combine them into a single object.
Pass window.location.toLocaleString() as the argument to apply to the current url.